之前写了个爬取 Github Trending 的服务 github-trending-api,因为网络原因,失败率比较高,最近在进行优化,会每个小时请求一次 Github,失败则重试5次,然后把成功的结果存到 Redis 中。记录下 Node.js 中操作 Redis 的一些方法。
¶一、安装 Redis
我这里是通过 Docker 来安装 Redis 的,具体可参考:前端学习 Docker 之旅(五)—— 安装 Redis 并启动、连接。
¶二、缓存类型
主要分为三种:数据库、本地应用缓存(内存等)、远程缓存(Redis),这里不展开细讲。
¶三、缓存模式
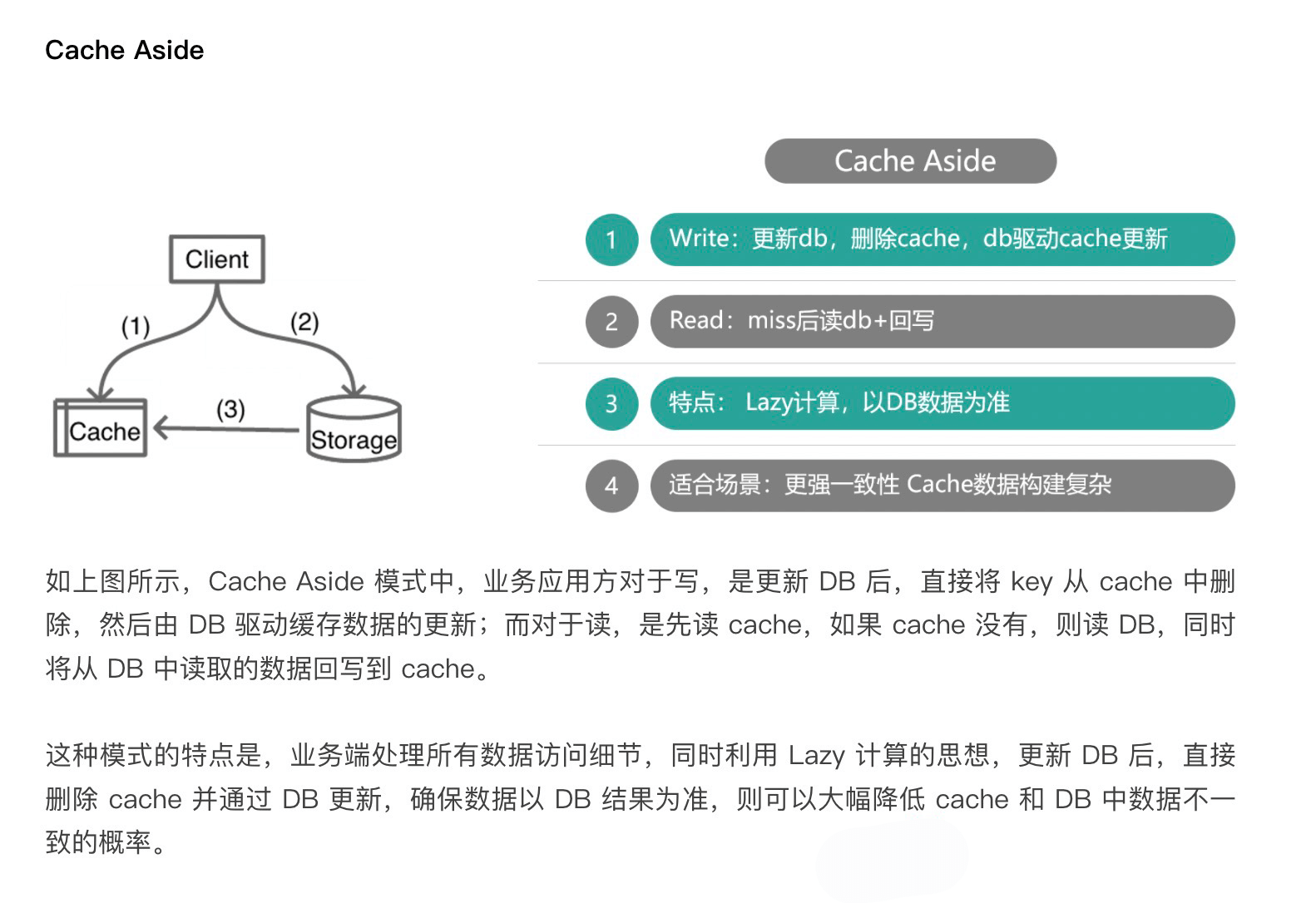
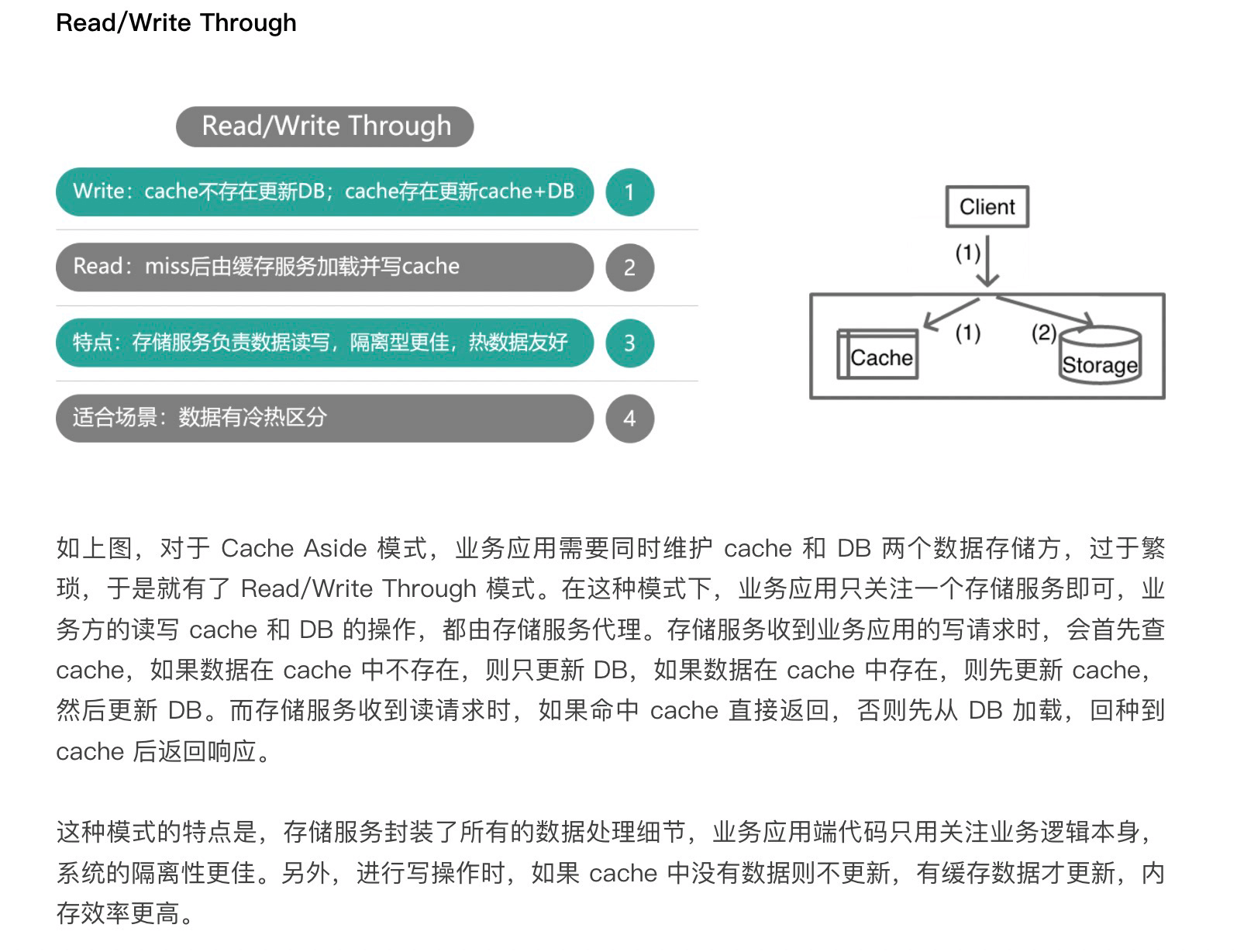
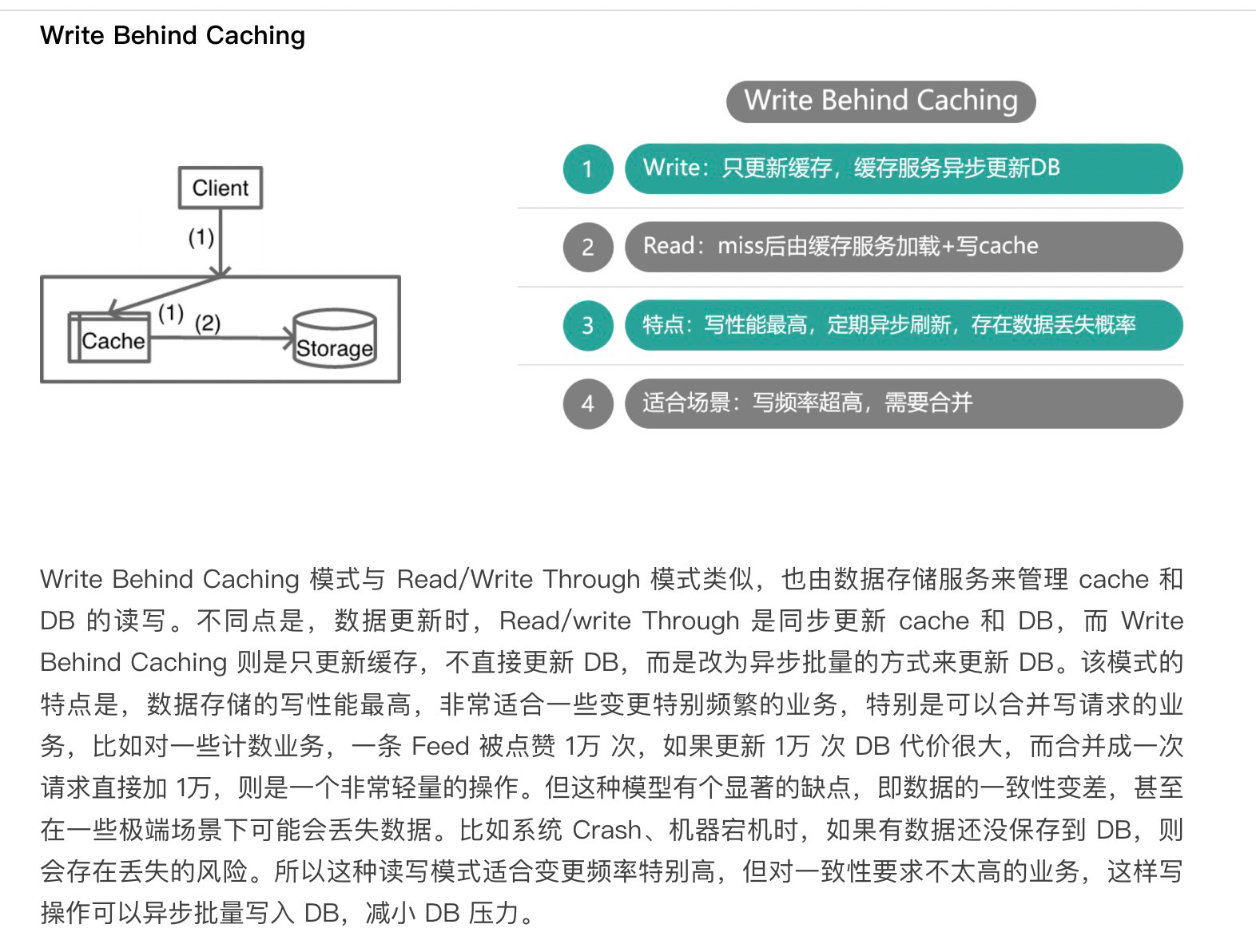
¶四、Node.js 与 Redis
1 | import { createClient } from 'redis'; |
语法:
1 | createClient({ |
¶五、Redis value 类型
Redis 的 key 是唯一的,如果 key 所对应的 value 是 string 类型,则不能再次覆盖修改为 hash 类型。点此查看其他方法
¶5.1、string
1 | await redis.set('key', 'value') |
不建议赋值后再设置过期时间,这样不能保证原子性。
¶5.2、hash
1 | const obj = { name: 'Tom' } |
取出存入 Redis 的对象时,每个 key 的值会被转成 string。
¶5.3、lists
¶5.4、sets
¶5.4、事务
1 | redis.multi() |
¶参考资料
1、nodejs操作redis总结
2、node-redis
3、https://redis.js.org/
4、涉及代码:https://github.com/liuxy0551/github-trending-api